Introduction
HTML, CSS and JavaScript are the three main building blocks that make the web run.
Using an analogy of a person, HTML could be described as the "bones" of the web, it provides the structure of every single webpage, CSS would be the clothes, as it makes the website look different, and JavaScript is like the muscles, as it allows the content to "move" and be interactive
HTML
HTML is an acronym for HyperText Markup Language
There are around 140 HTML elements.
To make a HTML page, you need the following tags:
html
- This tag is required to allow the browser to know that it is a HTML document.head
- This tag is needed to allow the browser to process the metadata about the webpage - it allows other tags, such asmeta
andtitle
to give the page and web crawlers informationbody
- This tag is where all the visible content of the website can be found.
HTML tags are not case-sensitive, so <html>
,
<HTML>
or even <hTmL>
will
work perfectly fine. However, it is
convention to create them in lowercase only
Here is an example HTML page:
!DOCTYPE html
html
<head
<title>
Page Title
</title</head
<body
<h1>
Hello, World!
</h1</body
/html
A tag can have attributes. These are pieces of information that the browser will use to change the behaviour of the tag
Here is an example of an attribute, from the <html>
tag:
html
lang=
"en"
In this instance, the lang
attribute tells the
browser that the
html page is in english or any other country code that could be there, for example fr
for France
There are other attributes that are useful:
p
and
id=
id-1
p
class=
class-1
Tags you must know for the exam:
-
html
-
head
-
link
-
title
body
-
h1
img
div
a
-
form
input
p
ul
ol
li
script
The a
tag
This is an Anchor tag, it allows hyperlinks to other documents
Here is the syntax:
<a href=
https://www.example.com/link/to/page
>Text To Display
/a
This would display as:
Text to DisplayIt, as all the other elements, would take in to account any CSS stylings
The img
tag
This tag embeds an image onto the webpage
It does not require a closing tag
Here is an example of the syntax
<img src=
https://via.placeholder.com/100x100.png
alt=
A Placeholder Image
width=
100
height=
200
>
This would render as:
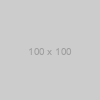
The alt
attribute is needed for
accessibility purposes, or in the event the image fails to load for any reason.
CSS
CSS is an acronym for Cascading Style Sheets. Each style "cascades" down to each sub element, unless it is overriden.
CSS is used to style webpages, and overall influence the look and feel
It can be applied in three ways: Inline, Embedded, and External
There are three ways that a style can be applied too: the HTML tag, a class, or an identifier (id)
Styles are written with a semicolon (;
) at the end of every
statement. This allows the file to be minified to make it small enough to be used in
production,
where timing is crucial.
Inline Styles
A style can be used directly in a html tag using the style=
attribute
key:value;
For example: <p
would render as:style=
>color:#f0f;
Hello, World
/p
Hello, World
Embedded Styles
Embedded Styles are within style
tags
These are written in the same way as inline, except they can be found without the attribute syntax so it would look like:
p {
color:
#fof
;
}
Convention says that there should be a space between the selector and {
however, this is not stricly needed
Embedded styles for a tag will apply to every one on the page
An inline style takes precedence over the embedded styles
External Stylesheets
A drawback to using embedded and inline stylesheets, is for a large website, it will be hard to maintain, and time wasting to use the same code again and again. So Stylesheets can be linked externally to allow for one change "cascading" throughout the entire site. This means that the dev can have an easier job, and the entire site can be changed in a few clicks.
An external stylesheet is linked using the link
tag
within the head
of the page using the
following
syntax:
<link rel=
stylesheet
href=
path/to/stylesheet.css
/>
External stylesheets can call others using the @import
syntax
CSS keys you must know
background-color
border-color
border-style
border-width
color
font-family
font-size
height
width
Notice that it uses the American spelling "color", not the British "Colour"
JavaScript
The Scripting Language of the web
An alternative to WASM (Web Assembly)
JavaScript can be found in two places: between script
tags, or linked externally through a
script.js
file.
JavaScript Syntax
JavaScript is a dynamically typed language, which means that it will automatically cast any variables to the correct type for the operation. For example:
let i = 1;
i--;
console.log(i==false);
//this will output true
This is unlike the static type languages such as Java or C#
To declare a variable in JavaScript, you can use
let i = 0;
var i = 0;
const i = 0;
const
defines a constant, a
variable
which
can never change.
let
and var
are slightly different from
eachother,
but
that is out of scope for the exam. (they are to do with the scope of the variable)
JavaScript can be used to interact with the DOM (the webpage)
You can use the following to select then modify a html element:
const element = document.getElementById("id"); //assumes you have an element with the id of id
element.innerHTML = "Hello world!" //This now changes the display from the element to "Hello World", or any text you put there.
JS is used for more than simple modifications, it can be used for buttons, such as
where the onclick
attribute
can
execute
simple JavaScript
For the exam, you will need to know how to make a HTML form, such as this:
All input is evil, until proven otherwise - Michael Howard (Microsoft's former Chief Software Architect)
You must always ensure that you validate your user input, as it is dangerous to send unvalidated code to any server. Without it, you could be at risk of XSS and SQL injections, which could have bad consequences.
To get the value of the form item, you can use:
const element = document.getElementById("id"); //assumes you have an element with the id of id
const value = element.value; // element.innerHtml if it is not in a form
JavaScript Runtimes
In JavaScript, semi-colons are optional, but conventional, as it is based on the Java programming language.
JavaScript is interpreted, not compiled for the same reason Java only compiles half way, "Write once, run anywhere" This is not entirely true, as not all js features are supported by all platforms
JavaScript is NOT an interpreted version of Java, the deprecated
applet
tag is used for that. They are no longer
related in
any
way!!
Read more about JavaScript here